Use of TypeScript type annotations
Overview of the tutorial
This tutorial covers how to start using the TomTom Maps SDK for Web with TypeScript type annotations.
Prerequisites
You will need the following:
- Node.js and npm installed
- API Key - If you don't have an API Key visit the How to get a TomTom API Key site and create one.
What are typings?
Typings are used to describe the format and structure of objects. With typings available, the compiler is able to investigate code and early discover errors like calling methods that don't exist on an object, or passing arguments of the wrong type.
Basic setup - JavaScript and downloadable package
This section covers the basic usage of type annotations in a JavaScript project with the TomTom Maps SDK for Web downloadable zip package.
Prerequisites
- TomTom Maps SDK for Web First, you need to download the Maps library from the downloads page.
- TypeScript
Then all you have to do is install the TypeScript via npm:
npm install -g typescript
Note that npm comes bundled with Node.js, so please make sure you have it installed on your machine.
Creating a project
Now we can create an empty project. Let's call it maps-with-typings-basic.
mkdir maps-with-typings-basiccd maps-with-typings-basic
With the empty project created, extract the dist directory from the Maps library zip package as web-sdk-maps and put it in the project root directory.
Setup
Now we need to create a tsconfig.json
file to make use of the typings.
The best way to do that is to use the tsc
command that comes with the TypeScript package.
tsc --init
It will generate a tsconfig.json file with some default settings and an extensive list of possible commented-out options.
By default TypeScript will only check the .ts files, and since we are using it only as a type
checker we need to uncomment the following options in the tsconfig.json
file:
"allowJs": true, /* Allow javascript files to be compiled. */
"checkJs": true, /* Report errors in .js files. */
"noEmit": true, /* Do not emit outputs. */
Code
Let's create an app.js
file where we will store our JavaScript code and index.html
where we
reference it along with the TomTom Maps SDK for Web.
Now copy this content into the index.html
file:
1<!DOCTYPE html>2<html class="use-all-space">3 <head>4 <meta http-equiv="X-UA-Compatible" content="IE=Edge" />5 <meta charset="UTF-8" />6 <title>My Map</title>7 <meta8 name="viewport"9 content="width=device-width,initial-scale=1,maximum-scale=1,user-scalable=no"10 />11 <link rel="stylesheet" type="text/css" href="./web-sdk-maps/maps.css" />12 <style>13 #map {14 width: 100vw15 height: 100vh;16 }17 </style>18 </head>19 <body>20 <div id="map" class="map"></div>21 <script src="./web-sdk-maps/maps-web.min.js"></script>22 <script src="./app.js"></script>23 </body>24</html>
To enable type checking for the TomTom Maps SDK for Web we have to reference the index.d.ts from the web-sdk-maps directory.
In order to do that, the first line of the app.js file must be in the following format:
/// <reference path="web-sdk-maps/index.d.ts" />
Now type checking is enabled and should be picked up by your IDE. If it's not please check if you have the proper extensions installed.
Advanced setup - JavaScript and Webpack + NPM
This section covers the more advanced use of type annotations where JavaScript, Webpack, and NPM are used.
Creating a project
Create an empty project. Let's call it maps-with-typings-js
1mkdir maps-with-typings-js2cd maps-with-typings-js3npm init -y
With the project initialized we can install the dependencies. First, we need to install the TypeScript package along with the Webpack as development dependencies.
npm install --save-dev typescript ts-loadernpm install --save-dev webpack webpack-cli webpack-dev-server css-loader style-loader
Then we can install the TomTom Maps SDK for Web:
npm install @tomtom-international/web-sdk-maps@next
Setup
First, we need to create a tsconfig.json
file. Use the tsc
command to do this:
tsc --init
After that we can create the Webpack configuration file webpack.config.js
with the following
content:
1var path = require("path")2module.exports = {3 entry: path.join(__dirname, "/app.js"),4 output: {5 filename: "bundle.js",6 path: __dirname,7 },8 module: {9 rules: [10 {11 test: /\.js$/,12 loader: "ts-loader",13 exclude: /node_modules/,14 },15 {16 test: /\.css$/,17 use: ["style-loader", "css-loader"],18 },19 ],20 },21 resolve: {22 extensions: [".ts", ".js"],23 },24}
Now let's add 'start' entry in scripts in the package.json file in order to run code:
1// ...2 "scripts": {3 "start": "webpack-dev-server --mode development"4 },5// ...
Code
We need to create 3 files to get this app running.
- index.html with the following content:
1<!DOCTYPE html>2<html class="use-all-space">3 <head>4 <meta http-equiv="X-UA-Compatible" content="IE=Edge" />5 <meta charset="UTF-8" />6 <title>My Map</title>7 <meta8 name="viewport"9 content="width=device-width,initial-scale=1,maximum-scale=1,user-scalable=no"10 />11 <link rel="stylesheet" type="text/css" href="./web-sdk-maps/maps.css" />12 <style>13 #map {14 width: 100vw;15 height: 100vh;16 }17 </style>18 </head>19 <body>20 <div id="map" class="map"></div>21 <script src="./bundle.js"></script>22 </body>23</html>
- style.css where we will import the TomTom Maps SDK for Web CSS style.
@import "@tomtom-international/web-sdk-maps";
- app.js is our main JavaScript file that will be bundled into the bundle.js file
1require("./style.css")2var tt = require("@tomtom-international/web-sdk-maps")34var map = tt.map({5 key: "<your-tomtom-API-Key>",6 container: "map",7})
Now we can start our app by running npm start
. Typings will be checked and errors will be
displayed in the console and in the IDE.
Advanced setup - TypeScript and Webpack + NPM
This section covers the more advanced use of type annotations where TypeScript, Webpack, and NPM are used.
Creating a project
Create an empty project. Let's call it maps-with-typings.
1mkdir maps-with-typings2cd maps-with-typings3npm init -y
With the project initialized we can install the dependencies. First, we need to install the TypeScript package along with the Webpack as development dependencies.
npm install --save-dev typescript ts-loadernpm install --save-dev webpack webpack-cli webpack-dev-server css-loader style-loader
Then we can install the TomTom Maps SDK for Web:
npm install @tomtom-international/web-sdk-maps@next
Setup
In the first place, we need to create a tsconfig.json
file. The best way to do that is by using
the tsc
command:
tsc --init
After that we can create the Webpack configuration file webpack.config.js
with the following
content:
1const path = require("path")2module.exports = {3 entry: path.join(__dirname, "/app.ts"),4 output: {5 filename: "app.js",6 path: __dirname,7 },8 module: {9 rules: [10 {11 test: /\.ts$/,12 loader: "ts-loader",13 exclude: /node_modules/,14 },15 {16 test: /\.css$/,17 use: ["style-loader", "css-loader"],18 },19 ],20 },21 resolve: {22 extensions: [".ts", ".js"],23 },24}
Now let's add 'start' entry in scripts in the package.json file in order to run code:
1// ...2 "scripts": {3 "start": "webpack-dev-server --mode development"4 },5// ...
Code
We need to create 3 files to get this app running.
- index.html with the following content:
1<!DOCTYPE html>2<html class="use-all-space">3 <head>4 <meta http-equiv="X-UA-Compatible" content="IE=Edge" />5 <meta charset="UTF-8" />6 <title>My Map</title>7 <meta8 name="viewport"9 content="width=device-width,initial-scale=1,maximum-scale=1,user-scalable=no"10 />11 <link rel="stylesheet" type="text/css" href="./web-sdk-maps/maps.css" />12 <style>13 #map {14 width: 100vw;15 height: 100vh;16 }17 </style>18 </head>19 <body>20 <div id="map" class="map"></div>21 <script src="./app.js"></script>22 </body>23</html>
- style.css where we will import the TomTom Maps SDK for Web CSS style.
@import "@tomtom-international/web-sdk-maps";
- app.ts our main TypeScript file that will be compiled into the app.js file
1import tt from "@tomtom-international/web-sdk-maps"2import "./style.css"34const map = tt.map({5 key: "<your-tomtom-API-Key>",6 container: "map",7})
Now we can start our app by running npm start
.
Usage - IDE and console
Let's put that code in our app.js / app.ts file and see what happens:
1tt.map({2 key: "<your-tomtom-API-Key>",3 container: "map",4 zoom: "eight",5})
As you can see, type checking is now working and detected that the 'zoom' property should be a number:
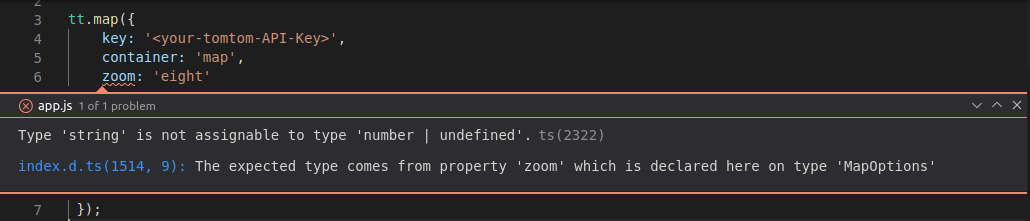
Alternatively, you can run the tsc
command from the console.
1~$ tsc2main.js:6:5 - error TS2322: Type 'string' is not assignable to type 'number | undefined'.36 zoom: 'eight'4 ~~~~5node_modules/@tomtom-international/web-sdk-maps/dist/index.d.ts:1514:96 1514 zoom?: number,7 ~~~~8 The expected type comes from property 'zoom' which is declared here on type 'MapOptions'9Found 1 error.
With TypeScript support you will also get the context-aware code completion feature:
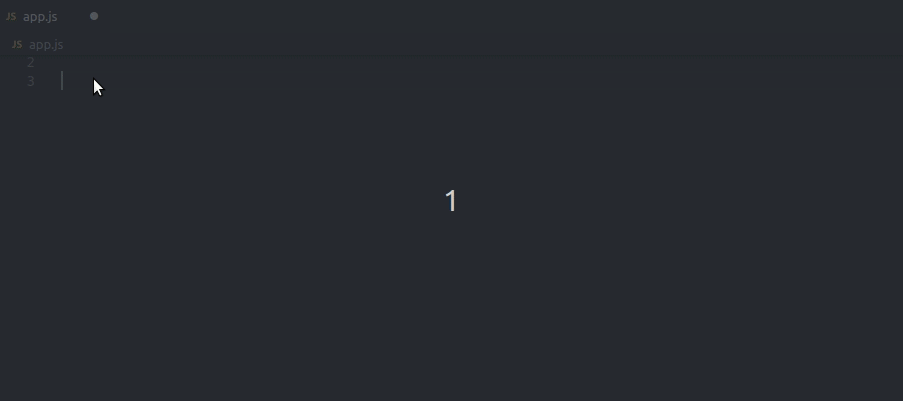